Tutorial: Request a quote
This tutorial shows you how to request a quote using the Liquidity Hub API. You will learn about the requests and responses your integration must handle to request a quote using the/quotes/request
endpoint.We use the example scenario of selling 1 Bitcoin (BTC) to buy US Dollars (USD). The quote you receive specifies the amount of USD you get in exchange for selling 1 BTC if you execute that quote.
Prerequisites
The/quotes/request
endpoint is protected by Bearer authentication. To successfully call this endpoint, you must include a valid Bearer authentication token in the request header. For more information, see the Get an authentication token tutorial.Construct the request body
ThePOST /quotes/request
operation accepts a body parameter where you specify the details of the trade you want to execute. The operation returns a quote that you can execute to complete the trade.In this tutorial, you want to sell 1 BTC in exchange for USD. Let's map this information to the fields in the request body:
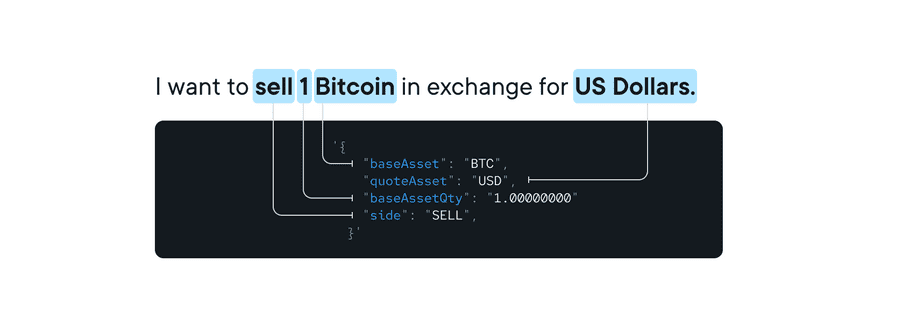
side
and asset quantity fields in the quote, see the Quote concept.Quote asset value
The
quoteAsset
value must be USD
. Requests with baseAsset
= USD
will result in an error response.Sample request
The following code sample requests a quote toSELL
1 BTC
in exchange for USD
:- Payload
- curl
application/json
{- "baseAsset": "BTC",
- "quoteAsset": "USD",
- "side": "SELL",
- "baseAssetQty": "1.00000000"
}
Examine the response
The code sample below shows the success response received from the/quotes/request
endpoint. The Quote
object in the response includes the bidPrice
, that is the quantity of USD you will receive by selling 1 BTC if you execute the quote. Let's take a closer look at some of the Quote
object fields:Field | Description |
---|---|
quoteId | The unique ID that identifies the quote. You need to specify this quoteId if you choose to execute this quote using the /quotes/execute endpoint. |
bidPrice | The quantity of quoteAsset (USD) you will receive in exchange for selling 1 unit of the baseAsset (BTC). |
offerPrice | This field is null because you are selling your baseAsset BTC. The offer price is specified only when the side = BUY , that is, when you want to buy the baseAsset in the trade. |
quoteAssetQty | The quantity of the quoteAsset (USD) you will receive if you execute the quote and sell 1 BTC. |
expiresAt | The time at which this quote becomes invalid. You cannot execute that quote after this time. |
For the full response object schema, see the Request a quote operation reference.
{- "quoteId": "fd5dbe64-a96e-4e1c-9843-75cd6b0cdb60",
- "requestedAt": "2021-01-01T01:00:30.000Z",
- "createdAt": "2021-01-01T01:00:32.000Z",
- "bidPrice": "51345.76",
- "offerPrice": null,
- "baseAsset": "BTC",
- "quoteAsset": "USD",
- "baseAssetQty": "1.00000000",
- "quoteAssetQty": "51345.76",
- "side": "SELL",
- "expiresAt": "2021-01-01T01:10:00.000Z",
- "endUserGuid": "669ce446-22d0-11ec-9621-0242ac130002",
- "fundSource": "FUNDED"
}
Error responses and handling
If your request is unsuccessful, you may receive a400
, 401
, 404
, or 500
error code from the Liquidity Hub API.Here are some recommendations for error handling:
Error code | Name | Recommended error handling |
---|---|---|
400 | Bad Request | Your request may be missing required header or body parameters. Inspect your request and retry the request. |
401 | Unauthorized | Your Bearer authentication token may be invalid or expired. Verify that the authentication token is not truncated or past the token expiry period. Retry the request again with a valid authentication token. |
403 | Forbidden | The base URL in your request could be incorrect. Retry the request with the correct base URL. |
404 | Not Found | No handler found for POST |
500 | Internal Server Error | The service is temporarily unavailable. Retry the request later. |
Next step: Execute the quote
Since every quote is valid for a limited time (specified by theexpiresAt
field in the response body), your integration should be able to quickly decide whether to execute a quote or not. As a best practice, specify price thresholds that the integration can compare the bidPrice
or offerPrice
against and decide whether the quote should be executed.If the price specified in the quote you received meets the criteria for completing the trade, your integration should call the /quotes/execute
endpoint to execute the quote. See the Execute a quote guide for more details.